-
Notifications
You must be signed in to change notification settings - Fork 8.5k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
wpf: add support for VT mouse mode (#5375)
## Summary of the Pull Request This pull request ports the VT mouse code from TermControl to WpfTerminalControl. Our WPF control is a lot closer to Win32 than to Xaml, so our mouse event handler looks _nothing_ like the one that we got from Xaml. We can pass events through almost directly, because the window message handling in the mouse input code actually came from _conhost_. It's awesome. Neither TermControl nor conhost pass hover events through when the control isn't focused, so I wired up focus events to make sure we acted the same. Just like Terminal and conhost, mouse events are suppressed when <kbd>Shift</kbd> is held. ## Validation Steps Performed Tested with MC, and tested by manually engaging SGR events in an Echo terminal. 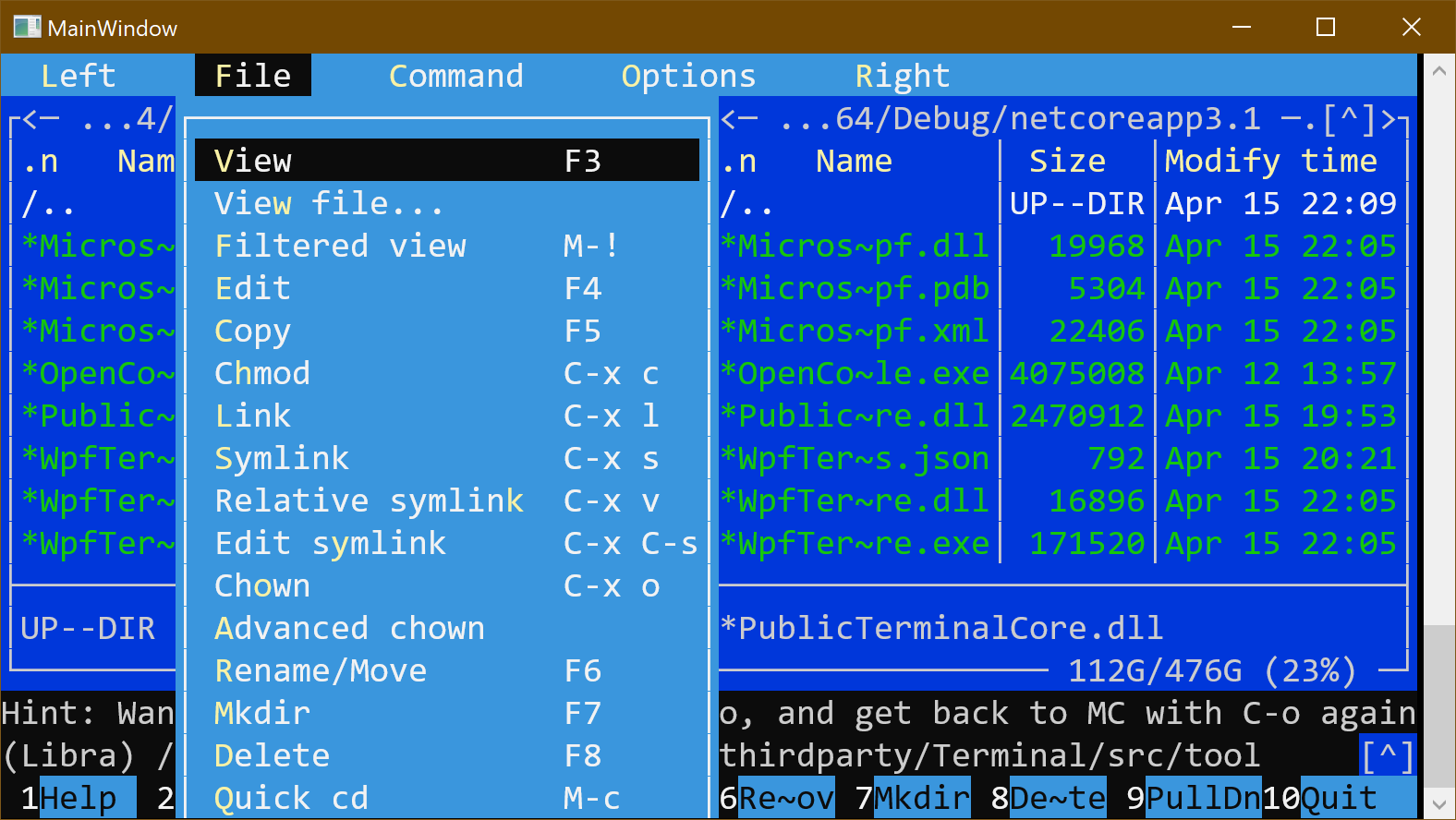
- Loading branch information
Showing
4 changed files
with
75 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters