Commit f987b18
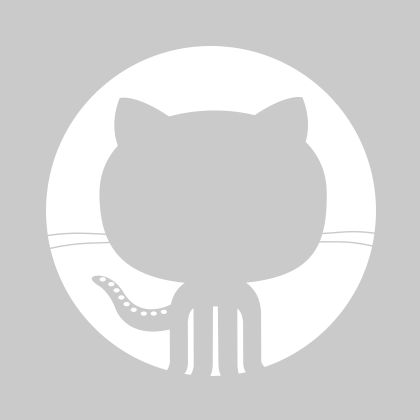
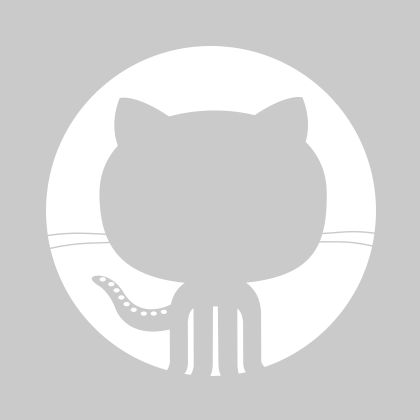
Marius Hanl
Jeanette Winzenburg
1 parent a272c4f commit f987b18
File tree
5 files changed
+138
-14
lines changed- modules/javafx.controls/src
- main/java/javafx/scene/control
- skin
- test/java/test/javafx/scene/control
5 files changed
+138
-14
lines changedOriginal file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
151 | 151 |
| |
152 | 152 |
| |
153 | 153 |
| |
| 154 | + | |
| 155 | + | |
| 156 | + | |
154 | 157 |
| |
155 | 158 |
| |
156 |
| - | |
| 159 | + | |
157 | 160 |
| |
158 | 161 |
| |
159 | 162 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
240 | 240 |
| |
241 | 241 |
| |
242 | 242 |
| |
243 |
| - | |
244 | 243 |
| |
| 244 | + | |
| 245 | + | |
245 | 246 |
| |
246 | 247 |
| |
247 | 248 |
| |
| |||
278 | 279 |
| |
279 | 280 |
| |
280 | 281 |
| |
281 |
| - | |
| 282 | + | |
| 283 | + | |
| 284 | + | |
| 285 | + | |
282 | 286 |
| |
283 | 287 |
| |
284 | 288 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
53 | 53 |
| |
54 | 54 |
| |
55 | 55 |
| |
| 56 | + | |
56 | 57 |
| |
57 | 58 |
| |
58 | 59 |
| |
| |||
321 | 322 |
| |
322 | 323 |
| |
323 | 324 |
| |
324 |
| - | |
325 |
| - | |
326 |
| - | |
| 325 | + | |
| 326 | + | |
| 327 | + | |
| 328 | + | |
| 329 | + | |
| 330 | + | |
| 331 | + | |
327 | 332 |
| |
328 | 333 |
| |
329 | 334 |
| |
| |||
396 | 401 |
| |
397 | 402 |
| |
398 | 403 |
| |
| 404 | + | |
| 405 | + | |
| 406 | + | |
| 407 | + | |
| 408 | + | |
399 | 409 |
| |
400 | 410 |
| |
401 | 411 |
| |
| |||
413 | 423 |
| |
414 | 424 |
| |
415 | 425 |
| |
416 |
| - | |
| 426 | + | |
417 | 427 |
| |
418 | 428 |
| |
419 | 429 |
| |
| |||
554 | 564 |
| |
555 | 565 |
| |
556 | 566 |
| |
| 567 | + | |
| 568 | + | |
| 569 | + | |
557 | 570 |
| |
558 |
| - | |
| 571 | + | |
559 | 572 |
| |
560 | 573 |
| |
561 | 574 |
| |
562 | 575 |
| |
563 |
| - | |
564 |
| - | |
565 |
| - | |
| 576 | + | |
| 577 | + | |
| 578 | + | |
| 579 | + | |
| 580 | + | |
| 581 | + | |
566 | 582 |
| |
567 | 583 |
| |
568 | 584 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 |
| - | |
| 2 | + | |
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
| |
| |||
26 | 26 |
| |
27 | 27 |
| |
28 | 28 |
| |
| 29 | + | |
29 | 30 |
| |
30 | 31 |
| |
31 | 32 |
| |
| |||
56 | 57 |
| |
57 | 58 |
| |
58 | 59 |
| |
59 |
| - | |
60 |
| - | |
61 | 60 |
| |
62 | 61 |
| |
63 | 62 |
| |
| |||
70 | 69 |
| |
71 | 70 |
| |
72 | 71 |
| |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
73 | 80 |
| |
74 | 81 |
| |
75 | 82 |
| |
76 | 83 |
| |
| 84 | + | |
| 85 | + | |
| 86 | + | |
| 87 | + | |
77 | 88 |
| |
78 | 89 |
| |
79 | 90 |
| |
| |||
154 | 165 |
| |
155 | 166 |
| |
156 | 167 |
| |
| 168 | + | |
| 169 | + | |
| 170 | + | |
| 171 | + | |
| 172 | + | |
| 173 | + | |
| 174 | + | |
| 175 | + | |
| 176 | + | |
| 177 | + | |
| 178 | + | |
| 179 | + | |
| 180 | + | |
157 | 181 |
| |
158 | 182 |
| |
159 | 183 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
285 | 285 |
| |
286 | 286 |
| |
287 | 287 |
| |
| 288 | + | |
| 289 | + | |
| 290 | + | |
| 291 | + | |
| 292 | + | |
| 293 | + | |
| 294 | + | |
| 295 | + | |
| 296 | + | |
| 297 | + | |
| 298 | + | |
| 299 | + | |
| 300 | + | |
| 301 | + | |
| 302 | + | |
| 303 | + | |
| 304 | + | |
| 305 | + | |
| 306 | + | |
| 307 | + | |
| 308 | + | |
| 309 | + | |
| 310 | + | |
| 311 | + | |
| 312 | + | |
| 313 | + | |
| 314 | + | |
| 315 | + | |
| 316 | + | |
| 317 | + | |
| 318 | + | |
| 319 | + | |
| 320 | + | |
| 321 | + | |
| 322 | + | |
| 323 | + | |
| 324 | + | |
| 325 | + | |
| 326 | + | |
| 327 | + | |
| 328 | + | |
| 329 | + | |
| 330 | + | |
| 331 | + | |
| 332 | + | |
| 333 | + | |
| 334 | + | |
| 335 | + | |
| 336 | + | |
| 337 | + | |
| 338 | + | |
| 339 | + | |
| 340 | + | |
| 341 | + | |
| 342 | + | |
| 343 | + | |
| 344 | + | |
| 345 | + | |
| 346 | + | |
| 347 | + | |
| 348 | + | |
| 349 | + | |
| 350 | + | |
| 351 | + | |
| 352 | + | |
| 353 | + | |
| 354 | + | |
| 355 | + | |
| 356 | + | |
| 357 | + | |
| 358 | + | |
| 359 | + | |
| 360 | + | |
| 361 | + | |
| 362 | + | |
| 363 | + | |
| 364 | + | |
288 | 365 |
| |
289 | 366 |
| |
290 | 367 |
| |
|
0 commit comments