|
| 1 | +# PSG AY-3-8910 BF MSX SDCC Library (fR3eL Project) |
| 2 | + |
| 3 | +``` |
| 4 | +Author: mvac7 |
| 5 | +Architecture: MSX |
| 6 | +Format: C Object (SDCC .rel) |
| 7 | +Programming language: C and Z80 assembler |
| 8 | +``` |
| 9 | + |
| 10 | + |
| 11 | +--- |
| 12 | +## Description |
| 13 | + |
| 14 | +This project is an opensource library functions for access to internal or external MSX PSG AY-3-8910. |
| 15 | + |
| 16 | +Designed for developing MSX applications using Small Device C Compiler (SDCC). |
| 17 | + |
| 18 | +It does not use the BIOS so it can be used to program both ROMs or MSX-DOS executables. |
| 19 | + |
| 20 | +This library is similar to the [PSG AY-3-8910 RT](https://github.com/mvac7/SDCC_AY38910RT_Lib), but instead of writing directly to the registers, |
| 21 | +it does so to a buffer that should be dumped to the AY in each VBLANK frame. |
| 22 | + |
| 23 | +It is designed to work together with the PT3player and/or ayFXplayer libraries, but you can also use it for your own or third-party player. |
| 24 | + |
| 25 | + |
| 26 | + |
| 27 | +It incorporates the SOUND function with the same behavior as the command included in MSX BASIC, |
| 28 | +as well as specific functions to modify the different sound parameters of the AY. |
| 29 | + |
| 30 | +Controls the I/O bits of register 7 (Mixer), of the internal AY. |
| 31 | + |
| 32 | +It allows to use the internal PSG of the MSX or an external one (like the one incorporated in the MEGAFLASHROM SCC+ or the Flashjacks). |
| 33 | + |
| 34 | +In the header file there is a definition of SWITCHER type, needed for the functions. |
| 35 | +This type uses the values "ON" or "OFF", which equals 1 and 0 respectively. |
| 36 | + |
| 37 | +Include definitions to improve the readability of your programs. |
| 38 | + |
| 39 | +In the source code (\examples), you can find applications for testing and learning purposes. |
| 40 | + |
| 41 | +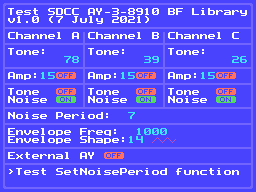 |
| 42 | + |
| 43 | +This library is part of the [MSX fR3eL Project](https://github.com/mvac7/SDCC_MSX_fR3eL). |
| 44 | + |
| 45 | +Enjoy it! |
| 46 | + |
| 47 | + |
| 48 | +--- |
| 49 | +## History of versions |
| 50 | + |
| 51 | +* v0.9b (07 July 2021) First version (Based in AY-3-8910 RT Library) |
| 52 | + |
| 53 | + |
| 54 | +--- |
| 55 | +## Requirements |
| 56 | + |
| 57 | +* Small Device C Compiler (SDCC) v4.1 > http://sdcc.sourceforge.net/ |
| 58 | +* Hex2bin v2.5 http://hex2bin.sourceforge.net/ |
| 59 | + |
| 60 | + |
| 61 | + |
| 62 | +--- |
| 63 | +## Definitions |
| 64 | + |
| 65 | + |
| 66 | +### AY Type |
| 67 | + |
| 68 | +Label | Value |
| 69 | +-- | -- |
| 70 | +AY_INTERNAL | 0 |
| 71 | +AY_EXTERNAL | 1 |
| 72 | + |
| 73 | + |
| 74 | +### AY Registers |
| 75 | + |
| 76 | +Label | Value | Description |
| 77 | +-- | -- | -- |
| 78 | +AY_ToneA | 0 | Channel A Tone Period (12 bits) |
| 79 | +AY_ToneB | 2 | Channel B Tone Period (12 bits) |
| 80 | +AY_ToneC | 4 | Channel C Tone Period (12 bits) |
| 81 | +AY_Noise | 6 | Noise Period (5 bits) |
| 82 | +AY_Mixer | 7 | Mixer |
| 83 | +AY_AmpA | 8 | Channel Volume A (4 bits + B5 active Envelope) |
| 84 | +AY_AmpB | 9 | Channel Volume B (4 bits + B5 active Envelope) |
| 85 | +AY_AmpC | 10 | Channel Volume C (4 bits + B5 active Envelope) |
| 86 | +AY_EnvPeriod | 11 | Envelope Period (16 bits) |
| 87 | +AY_EnvShape | 13 | Envelope Shape |
| 88 | + |
| 89 | + |
| 90 | +### Envelope shapes |
| 91 | + |
| 92 | +The header file defines envelope shapes in case you prefer to use it instead |
| 93 | +of the numerical form: |
| 94 | + |
| 95 | +Label | Value |
| 96 | +-- | -- |
| 97 | +AY_ENV_LowerBeat | 1 |
| 98 | +AY_ENV_Upper | 4 |
| 99 | +AY_ENV_LeftSaw | 8 |
| 100 | +AY_ENV_LowerTriangle | 10 |
| 101 | +AY_ENV_LowerHold | 11 |
| 102 | +AY_ENV_RightSaw | 12 |
| 103 | +AY_ENV_UpperHold | 13 |
| 104 | +AY_ENV_UpperTriangle | 14 |
| 105 | + |
| 106 | +**Attention!** The LowerBeat shape may be produced with the values: 0, 1, 2, 3 and 9. |
| 107 | +The value 1 has been assigned to *LowerBeat* as 0 can be useful in case you need to control when the envelope is triggered. In this case 0 can act as a "don't |
| 108 | +write the envelop" event. Remember that, every time the register 13 is written, the sound with the assigned shape is started. |
| 109 | + |
| 110 | +The Upper shape may be produced with the values: 4, 5, 6, 7 and 15. |
| 111 | + |
| 112 | + |
| 113 | + |
| 114 | +### AY channels |
| 115 | + |
| 116 | +You can use it in the functions: SetTonePeriod, SetVolume and SetChannel |
| 117 | + |
| 118 | +Label | Value |
| 119 | +-- | -- |
| 120 | +AY_Channel_A | 0 |
| 121 | +AY_Channel_B | 1 |
| 122 | +AY_Channel_C | 2 |
| 123 | + |
| 124 | + |
| 125 | +--- |
| 126 | +## Functions |
| 127 | + |
| 128 | +* **AY_Init**() - Initialize the buffer |
| 129 | +* **SOUND**(register, value) - Write into a register of PSG |
| 130 | +* **GetSound**(register) - Read PSG register value |
| 131 | +* **SetTonePeriod**(channel, period) - Set Tone Period for any channel |
| 132 | +* **SetNoisePeriod**(period) - Set Noise Period |
| 133 | +* **SetEnvelopePeriod**(period) - Set Envelope Period |
| 134 | +* **SetVolume**(channel, volume) - Set volume channel |
| 135 | +* **SetChannel**(channel, isTone, isNoise) - Mixer. Enable/disable Tone and Noise channels. |
| 136 | +* **SetEnvelope**(shape) - Set envelope type. Plays the sound on channels that have a volume of 16. |
| 137 | +* **PlayAY**() - Send data from AYREGS buffer to AY registers. (Execute on each interruption of VBLANK). |
| 138 | + |
| 139 | + |
| 140 | +--- |
| 141 | +## Set Internal or External AY |
| 142 | + |
| 143 | +To indicate in which PSG the sounds are to be played, you have the **AY_TYPE** variable. |
| 144 | +To select an external AY (ports 10h to 12h), like the one included in the MegaFlashROM SCC+, Flashjacks or other, you have to set the variable to 1 (or AY_EXTERNAL). |
| 145 | + |
| 146 | +``` |
| 147 | + AY_TYPE = AY_EXTERNAL; |
| 148 | +``` |
| 149 | + |
| 150 | +**Attention!** When you execute the AY_Init() function, it will be updated to the default value corresponding to the internal AY. |
| 151 | + |
| 152 | + |
| 153 | + |
| 154 | +## How to use this |
| 155 | + |
| 156 | +coming soon... |
| 157 | + |
| 158 | + |
| 159 | + |
| 160 | + |
| 161 | +--- |
| 162 | +## AY-3-8910 Register Table |
| 163 | + |
| 164 | +<table> |
| 165 | +<tr> |
| 166 | +<th colspan=2>Register\bit</th><th width=50>B7</th><th width=50>B6</th><th width=50>B5</th><th width=50>B4</th><th width=50>B3</th><th width=50>B2</th><th width=50>B1</th><th width=50>B0</th> |
| 167 | +</tr> |
| 168 | +<tr> |
| 169 | +<td>R0</td><td rowspan=2>Channel A Tone Period</td><td colspan=8 align=center>8-Bit Fine Tune A</td> |
| 170 | +</tr> |
| 171 | +<tr> |
| 172 | +<td>R1</td><td colspan=4></td><td colspan=4 align=center>4-Bit Coarse Tune A</td> |
| 173 | +</tr> |
| 174 | +<tr> |
| 175 | +<td>R2</td><td rowspan=2>Channel B Tone Period</td><td colspan=8 align=center>8-Bit Fine Tune B</td> |
| 176 | +</tr> |
| 177 | +<tr> |
| 178 | +<td>R3</td><td colspan=4></td><td colspan=4 align=center>4-Bit Coarse Tune B</td> |
| 179 | +</tr> |
| 180 | +<tr> |
| 181 | +<td>R4</td><td rowspan=2>Channel C Tone Period</td><td colspan=8 align=center>8-Bit Fine Tune C</td> |
| 182 | +</tr> |
| 183 | +<tr> |
| 184 | +<td>R5</td><td colspan=4></td><td colspan=4 align=center>4-Bit Coarse Tune C</td> |
| 185 | +</tr> |
| 186 | +<tr> |
| 187 | +<td>R6</td><td>Noise period</td><td colspan=3></td><td colspan=5 align=center>5-Bit Period control</td> |
| 188 | +</tr> |
| 189 | +<tr> |
| 190 | +<td rowspan=2>R7</td><td rowspan=2>Enable (bit 0=on, 1=off)</td><td colspan=2 align=center>IN/OUT</td><td colspan=3 align=center>Noise</td><td colspan=3 align=center>Tone</td></tr> |
| 191 | +<tr> |
| 192 | +<td align=center> IOB</td><td align=center>IOA</td><td align=center>C</td> |
| 193 | +<td align=center>B</td><td align=center>A</td><td align=center>C</td> |
| 194 | +<td align=center>B</td><td align=center>A</td> |
| 195 | +</tr> |
| 196 | +<tr> |
| 197 | +<td>R8</td><td>Channel A Amplitude</td><td colspan=2></td><td align=center>Env</td><td colspan=5 align=center>Amplitude</td> |
| 198 | +</tr> |
| 199 | +<tr> |
| 200 | +<td>R9</td><td>Channel B Amplitude</td><td colspan=2></td><td align=center>Env</td><td colspan=5 align=center>Amplitude</td> |
| 201 | +</tr> |
| 202 | +<tr> |
| 203 | +<td>R10</td><td>Channel C Amplitude</td><td colspan=2></td><td align=center>Env</td><td colspan=5 align=center>Amplitude</td> |
| 204 | +</tr> |
| 205 | +<tr> |
| 206 | +<td>R11</td><td rowspan=2>Envelope Period</td><td colspan=8 align=center>8-Bit Fine Tune Envelope</td> |
| 207 | +</tr> |
| 208 | +<tr> |
| 209 | +<td>R12</td><td colspan=8 align=center>8-Bit Coarse Tune Envelope</td> |
| 210 | +</tr> |
| 211 | +<tr> |
| 212 | +<td>R13</td><td>Envelope Shape/Cycle</td><td colspan=4></td><td>CONT</td><td>ATT</td><td>ALT</td><td>HOLD</td> |
| 213 | +</tr> |
| 214 | +<tr> |
| 215 | +<td>R14</td><td>I/O Port A Data Store</td><td colspan=8 align=center>8-Bit Parallel I/O on Port A</td> |
| 216 | +</tr> |
| 217 | +<tr> |
| 218 | +<td>R15</td><td>I/O Port B Data Store</td><td colspan=8 align=center>8-Bit Parallel I/O on Port B</td> |
| 219 | +</tr> |
| 220 | +</table> |
| 221 | + |
| 222 | + |
| 223 | +--- |
| 224 | +## Acknowledgments |
| 225 | + |
| 226 | +I want to give a special thanks to all those who freely share their knowledge with the MSX developer community. |
| 227 | + |
| 228 | +* Avelino Herrera > [(WEB)](http://msx.avelinoherrera.com) |
| 229 | +* Nerlaska > [(Blog)](http://albertodehoyonebot.blogspot.com.es) |
| 230 | +* Marq > [(Marq)](http://www.kameli.net/marq/) |
| 231 | +* MSXKun/Paxanga soft > [(WEB)](http://paxangasoft.retroinvaders.com/) |
| 232 | +* Fubukimaru [(gitHub)](https://github.com/Fubukimaru) |
| 233 | +* Andrear > [(Blog)](http://andrear.altervista.org/home/msxsoftware.php) |
| 234 | +* Sapphire/Z80ST > [(WEB)](http://z80st.auic.es/) |
| 235 | +* Fernando García > [(Curso)](http://www.z80st.es/cursos/bitvision-assembler) |
| 236 | +* Ramones > [(MSXblog)](https://www.msxblog.es/tutoriales-de-programacion-en-ensamblador-ramones/) - [(MSXbanzai)](http://msxbanzai.tni.nl/dev/faq.html) |
| 237 | +* Eric Boez > [(gitHub)](https://github.com/ericb59) |
| 238 | +* MSX Assembly Page > [(WEB)](http://map.grauw.nl/resources/msxbios.php) |
| 239 | +* Portar MSX Tech Doc > [(WEB)](https://problemkaputt.de/portar.htm) |
| 240 | +* MSX Resource Center > [(WEB)](http://www.msx.org/) |
| 241 | +* Karoshi MSX Community > [(WEB)](http://karoshi.auic.es/) |
| 242 | +* BlueMSX emulator >> [(WEB)](http://www.bluemsx.com/) |
| 243 | +* OpenMSX emulator >> [(WEB)](http://openmsx.sourceforge.net/) |
| 244 | +* Meisei emulator >> ? |
| 245 | + |
| 246 | + |
| 247 | +--- |
| 248 | +## References |
| 249 | + |
| 250 | +* [General Instrument AY-3-8910 (wikipedia)](https://en.wikipedia.org/wiki/General_Instrument_AY-3-8910) |
| 251 | +* [GI AY-3-8910 Datasheet (PDF)](http://map.grauw.nl/resources/sound/generalinstrument_ay-3-8910.pdf) |
0 commit comments