|
| 1 | +# rswitch |
| 2 | + |
| 3 | +The `rswitch` library provides a compact and flexible way to implement a switch-like functionality in TypeScript. It allows you to define cases and their corresponding actions using an object literal syntax. |
| 4 | + |
| 5 | +## Installation |
| 6 | + |
| 7 | +Install the `rswitch` library using npm: |
| 8 | + |
| 9 | +```bash |
| 10 | +npm install rswitch |
| 11 | +``` |
| 12 | + |
| 13 | +## Usage |
| 14 | + |
| 15 | +The `rswitch` function takes a key and an object containing cases and actions. It evaluates the key against the cases and returns the corresponding action. |
| 16 | + |
| 17 | +### Syntax |
| 18 | + |
| 19 | +```javascript |
| 20 | +rswitch(key, casesObj, options); |
| 21 | +``` |
| 22 | + |
| 23 | +- `key` : The value to evaluate against the cases. |
| 24 | +- `casesObj` : An object containing cases and their actions. |
| 25 | +- `options` : (Optional) An object to customize the behavior of the rswitch function. |
| 26 | + - `returnFunction` (optional, default: false): If set to `false`, the `rswitch` function will call actions that are functions and return their values. If set to `true`, the function will return the functions as is. |
| 27 | + |
| 28 | +### Example |
| 29 | + |
| 30 | +```javascript |
| 31 | +import { rswitch } from "rswitch"; |
| 32 | +// const {rswitch} = require("rswitch") // commonjs |
| 33 | + |
| 34 | +const result = rswitch( |
| 35 | + "dev", |
| 36 | + { |
| 37 | + designer: "Designer", |
| 38 | + "dev, web": "Freelancer", |
| 39 | + "": () => { |
| 40 | + console.log("Hello"); |
| 41 | + }, |
| 42 | + }, |
| 43 | + { |
| 44 | + returnFunction: true, |
| 45 | + } |
| 46 | +); |
| 47 | + |
| 48 | +console.log(result); |
| 49 | +// Output: Freelancer |
| 50 | +``` |
| 51 | + |
| 52 | +In this example, the `rswitch` function evaluates the key `'dev'` against the cases defined in `casesObj`. Since it matches the case `'dev, web'`, the corresponding action `'Freelancer'` is returned and assigned to the `result` variable. Finally, the value of `result` is logged to the console. |
| 53 | + |
| 54 | +### Case Definitions |
| 55 | + |
| 56 | +Cases are defined as key-value pairs in the `casesObj` object. |
| 57 | + |
| 58 | +- Single Case: `{ caseKey: action }` |
| 59 | +- Multiple Cases: `{ 'case1, case2, case3': action }` |
| 60 | +- Default Case: `{ '': action }` |
| 61 | + |
| 62 | +> Actions can be any value or a function that returns a value. If the action is a function, and the `options` object has `returnFunction` set to `false`, it is called, and the returned value is returned. |
| 63 | +
|
| 64 | +If no cases match the evaluated key, the `rswitch` function checks for a default case. If a default case is defined, its corresponding action is performed. If no default case is defined or its action is not provided, `undefined` is returned. |
| 65 | + |
| 66 | +<br/> |
| 67 | +If you'd like to contribute, please do submit a pull request. |
| 68 | + |
| 69 | +In case you want support my work |
| 70 | + |
| 71 | +[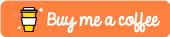](https://buymeacoffee.com/rashed.iqbal) |
0 commit comments