-
Notifications
You must be signed in to change notification settings - Fork 368
/
Copy pathRailfence.cpp
138 lines (118 loc) · 3.14 KB
/
Railfence.cpp
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
/*
# Railfence Cipher
Railfence cipher is a cryptographic technique which is a form of transposition cipher.
Given a plain-text message and a numeric key, cipher/de-cipher the given text using Rail Fence algorithm.
## Example:
Encryption
Input : "hello world"
Key = 3
Output : horel ollwd
Decryption
Input : horel ollwd
Key = 3
Output : hello world
## Note:
Initially The entire matrix is filled with "*"
## Ouput in c++ :
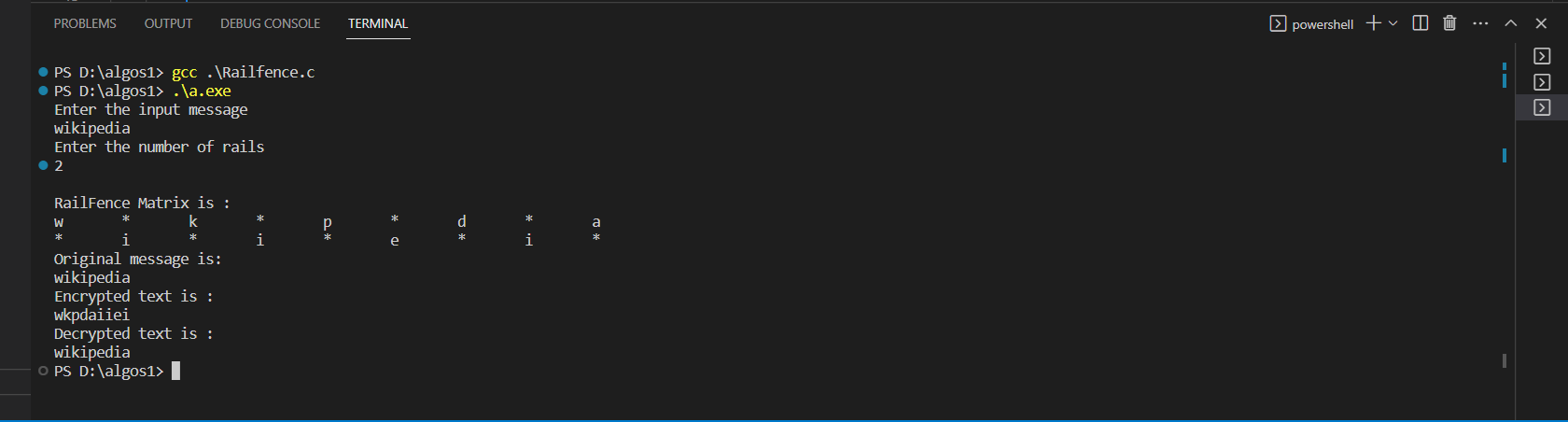
*/
#include <bits/stdc++.h>
using namespace std;
int main(){
string name;
int key,len;
//Enter the text u want to convert to railfence
cout<<"Enter the input message"<<endl;
cin>>name;
cout<<"Enter the key"<<endl;
cin>>key;
char matrix[30][30];
len = name.length();//len is the length of input message
//specify the number of rows/rails
for(int i=0;i<key;i++){
for(int j=0;j<len;j++){
matrix[i][j] = '*';
}
}
//code for obtaining railfence matrix
//length of input message is equal to the number of coloumns and number of rails equal to number of rows
int k=0,l=0,temp=0,flag=0;
//here the input message should be stored in railfence format.
for(int i=0;i<len;i++){
flag=0;
temp=0;
//if k value is equal to 0 or key-1 then convert the k value to negative so that it backtraverses the rows in railfence fashion.
if(k==0 || k==key-1){
k=k*(-1);
}
//since matrix index cannot be negative whenever k value is -ve convert to positive and after computation convert it back to original value
if(k<0){
temp=k;
k=k*(-1);
flag=1;
}
matrix[k][i]=name[l];
if(flag==1){
k=temp;
}
l++;
k++;
}
cout<<endl;
//printing the railfence matrix obtained.Railfence matrix even stores white spaces if the input message is a sentence.
printf("Railfence matrix is: \n");
for(int i=0;i<key;i++){
for(int j=0;j<len;j++){
cout<<" "<<matrix[i][j];
}
cout<<endl;
}
//code for encryption
char str[100];
int glo=0;
//string the encrypted message from railfence matrix if the entry of the matrix is not '*'
for(int i=0;i<key;i++){
for(int j=0;j<len;j++){
if(matrix[i][j] != '*'){
str[glo++]=matrix[i][j];
}
}
}
//printing original message
cout<<"original message is: "<<endl;
cout<<""<<name<<endl;
//printing encrypted message
cout<<"encrypted message is: "<<endl;
cout<<str<<endl;
char dstr[100];
int glob=0;
//code for decryption
cout<<"Decrypted message is: "<<endl;
int kk=0,temp1=0;
for(int i=0;i<len;i++){
temp1=0;
flag=0;
//if k value is equal to 0 or key-1 then convert the k value to negative so that it backtraverses the rows in railfence fashion.
if(kk==0 || kk==key-1){
kk=kk*(-1);
}
//since matrix index cannot be negative whenever k value is -ve convert to positive and after computation convert it back to original value
if(kk<0){
temp1=kk;
kk=kk*(-1);
flag=1;
}
//obtaining decrypted message from railfence matrix
char ct=matrix[kk][i];
dstr[glob++]=ct;
if(flag==1){
kk=temp1;
}
kk++;
}
//printing decrypted message
for(int i=0;dstr[i] !='\0';i++){
cout<<""<<dstr[i];
}
cout<<endl;
}